[Class structure playing card]
I recently went through a problem, where we need to make a class structure for a playing card problem in C# with 2 jokers.
Problem statement
We would like you to build us a deck of cards in a C# console app and do some normal card deck operations with this virtual set of cards, with 2 added jokers.
Jokers value witll be 0, Ace will be 1, Jack 11, Queen 12, King 13.
- Build us a deck of cards in a C# Or Node console application
- Populate the deck of cards with 54 cards: 4 suits and 2 jokers
- Display the cards on the console app
We will be building the application in the dot net c# and below is how we proceed.
Answer
- First open the VS Code, and open a new folder where you want to save the solution.
- Run this command in dot net CLI (Command Line Interface)
\src> dotnet new console
- Say Yes to everything, it will install dependencies to run the c# code.
- Create a new file in the solution namely PlayingCard.Cs, this will be your object model.
- Here we will be heaving our Enums for the Suites, and model to create Playing Cards, we will have a constructor with 2 params which will be Which Suit? and What Value?
Enum.cs
public enum Suites
{
Clubs,
Diamond,
Hearts,
Spades,
Joker
}
PlayingCards.cs
public class PlayingCards
{
public Suites Name { get; set; }
public int CardValue { get; set; }
public PlayingCards(int CardValue, Suites Name)
{
this.Name = Name;
this.CardValue = CardValue;
}
}
- Now, We will add the Deck.cs, this file will contain all the business operations like initialization of deck, shuffling and display etc.
Deck.cs
public class Deck
{
List<PlayingCards> lstPlayingCards = new List<PlayingCards>();
public void FillDeck()
{
//13*4 => 52
//Ace 1, Jack 11, Q=>12, K=>13
//Joker = 0
for (int i = 0; i < 52; i++)
{
//Getting suite value by taking the divided value
Suites s = (Suites)Math.Floor(Convert.ToDecimal(i / 13));
//Adding 1 to remainder as the card value starts with 1
int cardValue = i % 13 + 1;
PlayingCards pc = new PlayingCards(cardValue, s);
lstPlayingCards.Add(pc);
}
PlayingCards J1 = new PlayingCards(0, Suites.Joker);
lstPlayingCards.Add(J1);
PlayingCards J2 = new PlayingCards(0, Suites.Joker);
lstPlayingCards.Add(J2);
}
public void PrintDeck()
{
foreach (PlayingCards p in lstPlayingCards)
{
Console.WriteLine(FormatCards(p));
}
}
private string FormatCards(PlayingCards p)
{ //Ace 1, Jack 11, Q=>12, K=>13
//Joker = 0
switch (p.CardValue)
{
case 0:
return "Joker ";
case 1:
return "Ace of " + p.Name.ToString();
case 11:
return "Jack of " + p.Name.ToString();
case 12:
return "Queen of " + p.Name.ToString();
case 13:
return "King of " + p.Name.ToString();
default:
return p.CardValue + " of " + p.Name.ToString();
}
}
- The above code has three different functions, two are public which basically do initialization and printing. The third function is to format the output.
- Make sure to read the comments in the code to know why that particular calculation is done.
- Use them like below in your program.cs
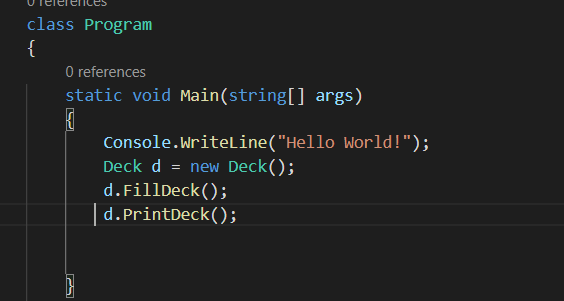
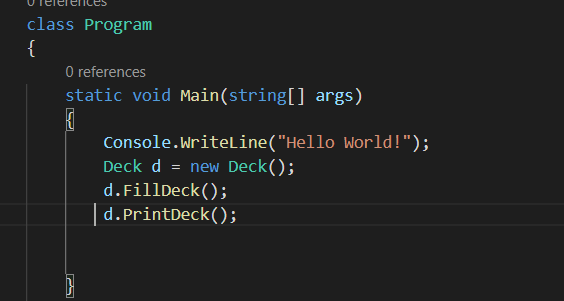
Output in terminal by running following command
\src> dotnet build
\src> dotnet run
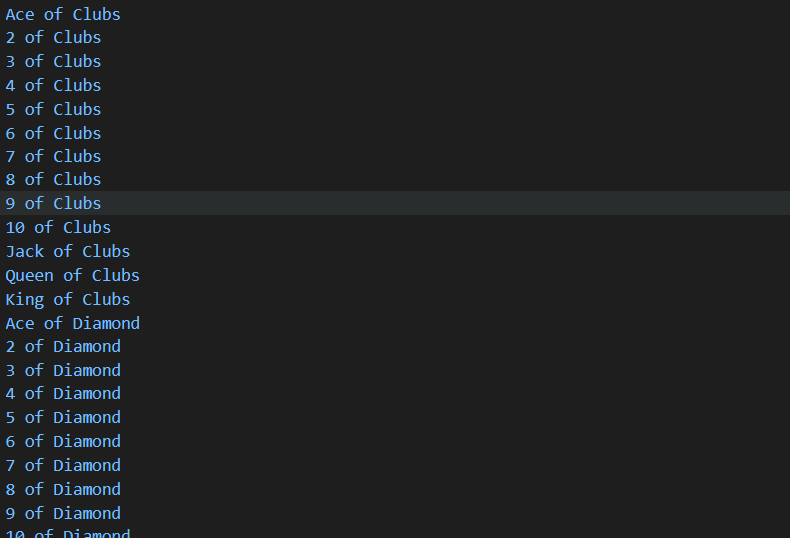
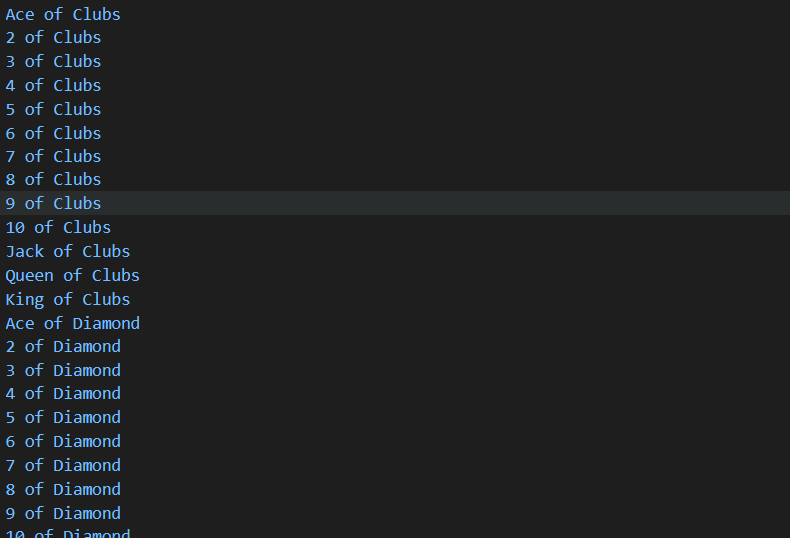
So this is how you create a class structure for playing card problem in C#.
Happy Coding.
Read Dependency Injection in c#:
FAQ: Class structure for playing card problem in C#
Visual Studio Code combines the simplicity of a source code editor with powerful developer tooling, like IntelliSense code completion and debugging. See More.
As per Wiki: A standard 52-card deck comprises 13 ranks in each of the four French suits: clubs (♣), diamonds (♦), hearts (♥) and spades (♠), with reversible (double-headed) court cards (face cards).
Add 1 to remainder by dividing the loop index with 13
int cardValue = i % 13 + 1;
Because the card value starts with 1 which is an Ace.
Create the class as mentioned above, this way both becomes part of one single entity (Encapsulation) and Get suite by taking the divided integer value of loop index with 13.
Suites s = (Suites)Math.Floor(Convert.ToDecimal(i / 13));
Recent Posts:
Pingback: DropBox - System Design - Thought Gem
Pingback: Race Conditions in Distributed or Multithreaded System - Thought Gem